Develop an interface for interacting with a database
Develop NetLogics that: insert values into the database, query the database, and display the query results.
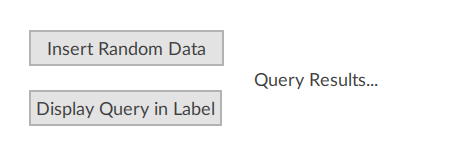
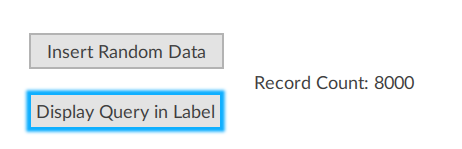
Prerequisites
To develop the project
-
Select
Run to run the project using the client emulator to test your project. To run the project on a remote client, see Add a client device.
-
Expand the project by adding more interface elements and
Insert
,Update
,Delete
, andSelect
methods.
You can download a sample project from: InteractWithDatabaseExpanded.zip[ExportMethod] public void Insert(int value) { var values = new object[1,2]; values[0,0] = DateTime.Now; values[0,1] = value; myTable.Insert(dbColumns, values); Log.Info("Insert","Inserted value: " + value.ToString()); } [ExportMethod] public void Update(int value, DateTime timestamp) { Object[,] ResultSet; String[] Header; myStore.Query("UPDATE Demo SET Value = " + value + " WHERE Timestamp = \"" + timestamp.ToString("o", CultureInfo.InvariantCulture) + "\"" , out Header, out ResultSet); Log.Info("Update", "Updated last record"); } [ExportMethod] public void Delete(int value) { Object[,] ResultSet; String[] Header; myStore.Query("DELETE FROM Demo WHERE Value<=65535 ORDER BY Timestamp DESC LIMIT 1", out Header, out ResultSet); Log.Info("Delete", "Deleted last record"); } [ExportMethod] public void Select(out int value) { Object[,] ResultSet; String[] Header; myStore.Query("SELECT * FROM Demo ORDER BY Timestamp DESC LIMIT 1", out Header, out ResultSet); value = Convert.ToInt32(ResultSet[0,1]); }