Develop the subscriber NetLogic and interface
Develop a NetLogic and integrate it with the interface.
Develop the NetLogic
- Right-click MainWindow (type) and select .
-
Hover-over the NetLogic, select
, and enter SubscriberLogic.
-
Double-click the NetLogic.
The external code editor opens.
-
In the code editor, replace the existing code with the following code:
#region StandardUsing using System; using FTOptix.CoreBase; using FTOptix.HMIProject; using UAManagedCore; using OpcUa = UAManagedCore.OpcUa; using FTOptix.NetLogic; using FTOptix.UI; using FTOptix.OPCUAServer; #endregion using uPLibrary.Networking.M2Mqtt; using uPLibrary.Networking.M2Mqtt.Messages; public class SubscriberLogic : BaseNetLogic { public override void Start() { var brokerIpAddressVariable = Project.Current.GetVariable("Model/BrokerIpAddress"); // Create a client connecting to the broker (default port is 1883) subscribeClient = new MqttClient(brokerIpAddressVariable.Value); // Connect to the broker subscribeClient.Connect("SubscriberClient"); // Assign a callback to be executed when a message is received from the broker subscribeClient.MqttMsgPublishReceived += SubscribeClientMqttMsgPublishReceived; // Subscribe to the "my_topic" topic with QoS 2 ushort msgId = subscribeClient.Subscribe(new string[] { "/my_topic" }, // topic new byte[] { MqttMsgBase.QOS_LEVEL_EXACTLY_ONCE }); // QoS level messageVariable = Project.Current.GetVariable("Model/Message"); } public override void Stop() { subscribeClient.Unsubscribe(new string[] { "/my_topic" }); subscribeClient.Disconnect(); } private void SubscribeClientMqttMsgPublishReceived(object sender, MqttMsgPublishEventArgs e) { messageVariable.Value = "Message received: " + System.Text.Encoding.UTF8.GetString(e.Message); } private MqttClient subscribeClient; private IUAVariable messageVariable; }
- Save the code.
Create the interface elements
-
Create the message variable by performing the following actions:
- In Project view, right-click Model and select .
-
Hover-over the variable, select
, and enter Message.
- In Properties, select Int32 and select String.
-
Create the Subscribe label by performing the following actions:
- Arrange the interface elements.
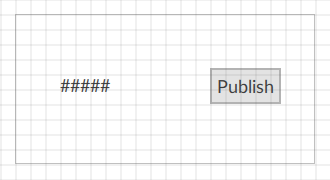